使用HTML CSS和JavaScript设计响应式滑动登录与注册表单
在本文中,我们将学习如何使用HTML、CSS和JavaScript创建响应式滑动登录与注册表单,并通过示例理解其实现方式。
现在许多网站都使用滑动登录与注册表单。注册表单用于为新用户注册或创建新账户,登录表单用于输入已注册用户的详细信息进入其个人账户或门户。因此,许多网站在两个不同页面上实现这两种方法。在本文中,我们将学习如何将这两种表单合并到一个HTML页面中,表单将根据用户的选择显示。在表单的顶部,一个按钮将帮助用户根据自己的选择选择登录或注册表单。当用户切换一个表单到另一个表单时,表单将显示流畅的滑动动画。
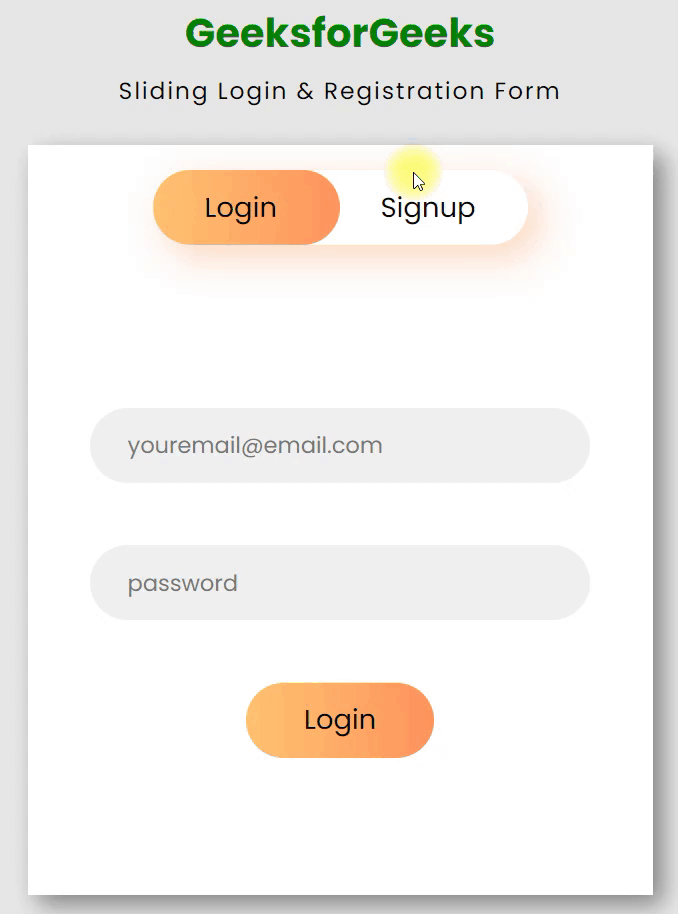
方法: 将使用以下方法创建响应式滑动登录和注册表单:
- 创建一个项目文件夹,在其中创建三个文件:“ index.html ”(用于编写HTML代码),“ style.css ”(用于编写CSS代码)和“ index.js ”(用于编写js代码)。 你还可以创建一个单独的文件,来存放该页面的响应式CSS代码。
- 现在,创建一个头部部分,用于添加标题。然后,在头部部分的下方创建一个“ container ”div,用于容纳完整的登录和注册表单,以及表示切换按钮。
- 接下来,创建一个div,用于放置两个切换表单的按钮,并在该div里创建两个按钮。一个按钮用于“ 登录 ”,另一个按钮用于“ 注册 ”。然后,在按钮div的上方创建一个 slider div,当某人点击相应的按钮时,它会改变其位置。 将按钮div的 margin-left 和 margin-right 设置为“ auto ”。然后,你会看到按钮会处于中心位置,添加CSS代码来去掉边框和轮廓,并使按钮div变为 flex 。请按照以下CSS代码来实现它:
- 现在,给滑块(div)添加背景颜色,并使其与按钮(div)具有相同的高度,宽度应为按钮(div)的一半。然后将 position absolute 和 z-index 设置为1。然后添加left和top以使div与按钮(div)的起始位置对齐。它应该覆盖按钮(div)的前半部分。此外,添加0.5秒的 transition 动画效果,以实现滑块移动时的平滑动画效果。
- 现在,在CSS中添加一个附加的类,当有人点击第二个按钮时会添加该类,在其中添加左对齐,使滑块覆盖按钮(div)的后半部分。
- 现在,编写javascript代码根据用户的选择移动滑块按钮。对“注册”按钮添加点击事件的监听器, addEventListener ,并添加在上一步中已在CSS中定义的类。然后,对“登录”按钮添加点击事件以移除该类(使用 classList .add(“class_name”) 添加类,使用 classList .remove(“class_name”) 移除类)。
- 像滑块按钮一样,我们将使用相同的方法创建滑动表单。在按钮(div)下面,我们将创建另一个部分或div,其中将包含两个表单。在此表单(div)中,我们将创建两个div。一个包含登录表单,另一个包含注册表单。然后在登录和注册区域内创建所需字段以及登录和注册按钮。使登录和注册区域的宽度等于容器div的宽度,表单区域div的宽度为容器div的两倍。
- 将表单区域设置为“ display: flex; position: relative; left: 0px; ”。在容器div中添加“ overflow: hidden; ”来隐藏超出容器之外的第二个表单。现在在CSS中编写一个额外的类,当有人点击第二个按钮时会添加该类,并在其中创建左对齐,使表单向左滑动,第二个表单将可见。
- 现在在javascript中编写代码,当有人点击注册按钮时,将额外的类(上一步中在CSS中已定义)添加到表单部分中,并在登录按钮被点击时删除该类。
示例: 这个示例演示了如何使用HTML、CSS和Javascript构建一个响应式的滑动登录和注册表单。
HTML
CSS
@import url(
"https://fonts.googleapis.com/css2?family=Poppins:ital,wght@0,100;0,200;0,300;0,400;0,500;0,600;0,700;0,800;0,900;1,100;1,200;1,300;1,400;1,500;1,600;1,700;1,800;1,900&display=swap");
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Poppins", sans-serif;
}
body {
height: 100vh;
width: 100vw;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
gap: 30px;
background-color: rgb(231, 231, 231);
}
header {
width: 100%;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
gap: 8px;
}
.heading {
color: green;
}
.title {
font-weight: 400;
letter-spacing: 1.5px;
}
.container {
height: 600px;
width: 500px;
background-color: white;
box-shadow: 8px 8px 20px rgb(128, 128, 128);
position: relative;
overflow: hidden;
}
.btn {
height: 60px;
width: 300px;
margin: 20px auto;
box-shadow: 10px 10px 30px rgb(254, 215, 188);
border-radius: 50px;
display: flex;
justify-content: space-around;
align-items: center;
}
.login,
.signup {
font-size: 22px;
border: none;
outline: none;
background-color: transparent;
position: relative;
cursor: pointer;
}
.slider {
height: 60px;
width: 150px;
border-radius: 50px;
background-image: linear-gradient(to right,
rgb(255, 195, 110),
rgb(255, 146, 91));
position: absolute;
top: 20px;
left: 100px;
transition: all 0.5s ease-in-out;
}
.moveslider {
left: 250px;
}
.form-section {
height: 500px;
width: 1000px;
padding: 20px 0;
display: flex;
position: relative;
transition: all 0.5s ease-in-out;
left: 0px;
}
.form-section-move {
left: -500px;
}
.login-box,
.signup-box {
height: 100%;
width: 500px;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
padding: 0px 40px;
}
.login-box {
gap: 50px;
}
.signup-box {
gap: 30px;
}
.ele {
height: 60px;
width: 400px;
outline: none;
border: none;
color: rgb(77, 77, 77);
background-color: rgb(240, 240, 240);
border-radius: 50px;
padding-left: 30px;
font-size: 18px;
}
.clkbtn {
height: 60px;
width: 150px;
border-radius: 50px;
background-image: linear-gradient(to right,
rgb(255, 195, 110),
rgb(255, 146, 91));
font-size: 22px;
border: none;
cursor: pointer;
}
@media screen and (max-width: 650px) {
.container {
height: 600px;
width: 300px;
}
.title {
font-size: 15px;
}
.btn {
height: 50px;
width: 200px;
margin: 20px auto;
}
.login,
.signup {
font-size: 19px;
}
.slider {
height: 50px;
width: 100px;
left: 50px;
}
.moveslider {
left: 150px;
}
.form-section {
height: 500px;
width: 600px;
}
.form-section-move {
left: -300px;
}
.login-box,
.signup-box {
height: 100%;
width: 300px;
}
.ele {
height: 50px;
width: 250px;
font-size: 15px;
}
.clkbtn {
height: 50px;
width: 130px;
font-size: 19px;
}
}
@media screen and (max-width: 320px) {
.container {
height: 600px;
width: 250px;
}
.heading {
font-size: 30px;
}
.title {
font-size: 10px;
}
.btn {
height: 50px;
width: 200px;
margin: 20px auto;
}
.login,
.signup {
font-size: 19px;
}
.slider {
height: 50px;
width: 100px;
left: 27px;
}
.moveslider {
left: 127px;
}
.form-section {
height: 500px;
width: 500px;
}
.form-section-move {
left: -250px;
}
.login-box,
.signup-box {
height: 100%;
width: 250px;
}
.ele {
height: 50px;
width: 220px;
font-size: 15px;
}
.clkbtn {
height: 50px;
width: 130px;
font-size: 19px;
}
}
JavaScript
输出: 从输出中,您还可以看到网站的响应性。
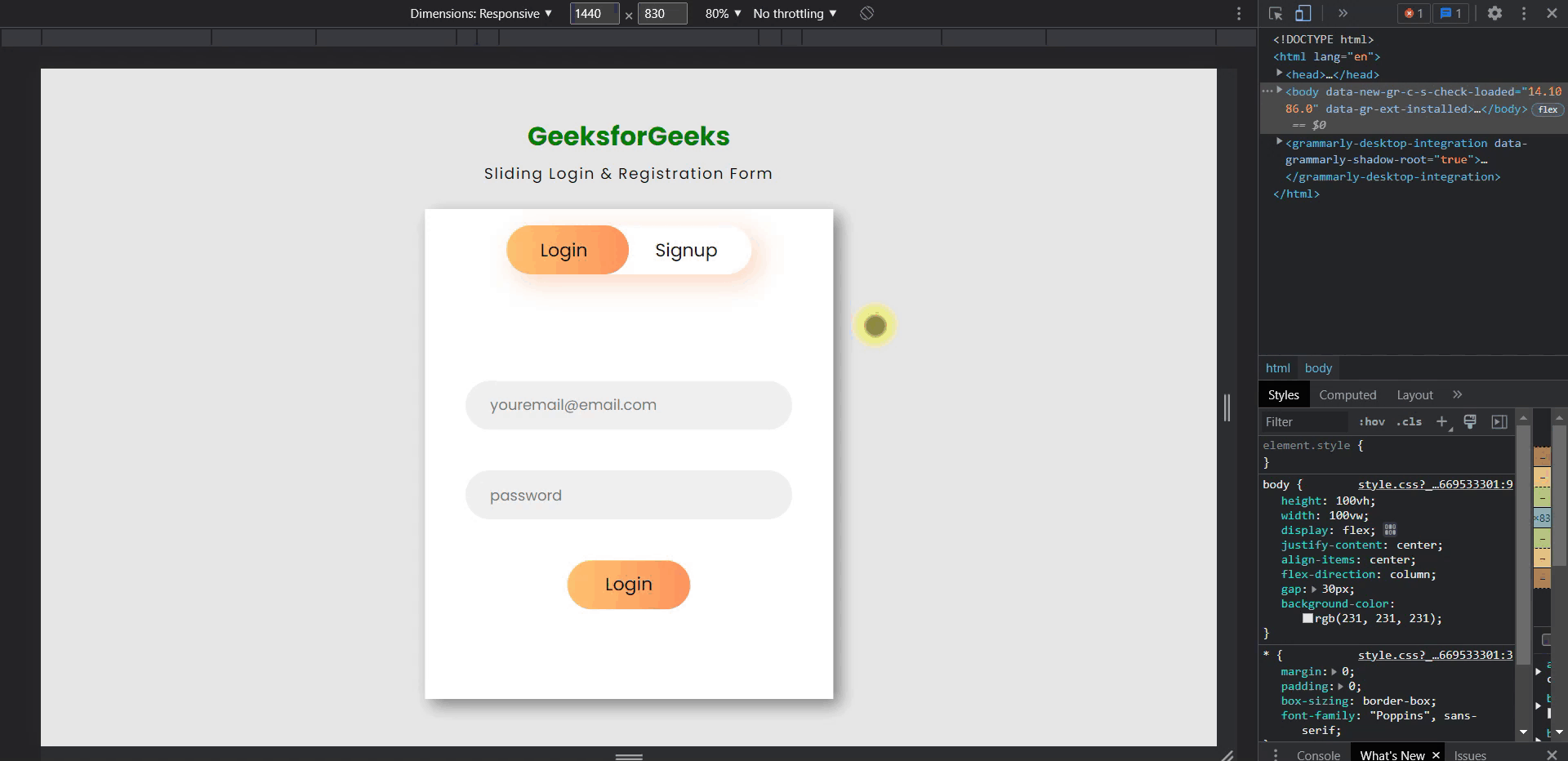