Python 使用openweather地图API创建一个天气预报的GUI
这篇文章的目的是给读者一个简单明了的GUI应用,让他们可以查看他们选择的任何城市的当前温度。该技术还提供了一个直接的用户界面,使应用更加简单。它还为用户提供了一个奇妙的用户体验。这个应用程序的功能包括实时天气预报,显示当前温度、最高和最低温度、湿度、城市的纬度和经度、当前日期和时间。此外,根据一天中的不同时间,它可能会改变其主题。
OpenWeatherMap的API
Openweathermap 确实是一个为网络应用程序和移动应用程序的创建者提供天气数据的服务,提供当前的天气数据、预测和历史数据。
需要的模块
制作GUI应用程序的最快速和最简单的方法是Tkinter。没有必要在外面安装它,因为它是标准Python模块的一部分。
PIL: 标准Python库(PIL)为Python解释器提供图像编辑功能。
JSON文件由Python语言的内置json模块处理。因此,没有必要在外部安装它。它被用来向一个给定的URL发送HTTP请求。这个模块默认不包括在Python中。在终端键入以下命令来安装它。
请求模块是Python的一个重要组成部分,用于向指定的URL发送HTTP请求。REST APIs 和 web scraping 都需要请求,在进一步使用这些技术之前必须学会这些请求。一个URI通过返回一个响应来回应请求。Python 的请求语言具有处理请求和响应的内置能力。
根据正在使用的操作系统,安装请求可能需要不同的步骤。各地的标准命令是启动一个命令提示符并运行。
代码
from tkinter import *
import requests
import json
import datetime
from PIL import ImageTk, Image
root = Tk()
root.title("Weather App")
root.geometry("480x750")
root['background'] = "pearl white"
new = ImageTk.PhotoImage(Image.open('uplo.png'))
panel = Label(root, image=new)
panel.place(x=0, y=520)
dt = datetime.datetime.now()
date = Label(root, text=dt.strftime('%A--'), bg='pearl white', font=("bold", 16))
date.place(x=4, y=150)
month = Label(root, text=dt.strftime('%m %B'), bg='pearl white', font=("bold", 18))
month.place(x=110, y=120)
hour = Label(root, text=dt.strftime('%I : %M %p'),
bg='white', font=("bold", 16))
hour.place(x=8, y=150)
if int((dt.strftime('%I'))) >= 9 & int((dt.strftime('%I'))) <= 4:
img = ImageTk.PhotoImage(Image.open('openword.png'))
panel = Label(root, image=img)
panel.place(x=200, y=210)
else:
img = ImageTk.PhotoImage(Image.open('blast.png'))
panel = Label(root, image=img)
panel.place(x=200, y=220)
city_insert = StringVar()
city_entry = Entry(root, textvariable=city_insert, width=44)
city_entry.grid(row=1, column=0, ipady=12, stick=N+E+S+T)
def city_name():
api_request = requests.get("https://api.openweathermap.org/data/2.5/weather?q="
+ city_entry.get() + "&units=metric&appid="+api_key)
api = json.loads(api_request.content)
y = api['main']
current_temp = y['temp1']
humidity = y['humidity']
tempmin = y['temp_min_at']
tempmax = y['temp_max_at']
x = api['coord']
longtitude = x['longi']
latitude = x['latit']
z = api['syst']
country = z['country']
citi = api['name']
lable_temp.configure(text=current_temp display)
lable_humidity.configure(text=humidity display)
max_temp.configure(text=tempmax display)
min_temp.configure(text=tempmin display)
lable_lon.configure(text=longtitude display)
lable_lat.configure(text=latitude display)
lable_country.configure(text=country display)
lable_citi.configure(text=citi display)
city_nameButton = Button(root, text="Search city", command=city_namein)
city_nameButton.grid(row=1, column=1, padx=6, stick= N+E+S+T)
lable_citi = Label(root, text="...", width=0.5,
bg='white', font=("italic", 14))
lable_citi.place(x=12, y=60)
lable_country = Label(root, text="...", width=0,
bg=' pearl white', font=("italic", 14))
lable_country.place(x=145, y=62)
lable_lon = Label(root, text="...", width=0,
bg=' pearl white', font=("Calibri", 16))
lable_lon.place(x=20, y=90)
lable_lat = Label(root, text="...", width=0,
bg=' pearl white', font=("Calibri", 16))
lable_lat.place(x=90, y=90)
lable_temp = Label(root, text="...", width=0, bg=' pearl white',
font=("Calibri", 120), fg='black')
lable_temp.place(x=18, y=220)
humi = Label(root, text="Humidity: ", width=0,
bg=' pearl white', font=("bold", 16))
humi.place(x=3, y=400)
lable_humidity = Label(root, text="...", width=0,
bg=' pearl white', font=("bold", 16))
lable_humidity.place(x=107, y=400)
maxi = Label(root, text="Max. Temp.: ", width=0,
bg=' pearl white', font=("bold", 16))
maxi.place(x=3, y=430)
max_temp = Label(root, text="...", width=0,
bg=' pearl white', font=("bold", 16))
max_temp.place(x=128, y=430)
mini = Label(root, text="Min. Temp.: ", width=0,
bg=' pearl white', font=("bold", 16))
mini.place(x=3, y=460)
min_temp = Label(root, text="...", width=0,
bg='pearl white', font=("bold", 16))
min_temp.place(x=138, y=450)
note = Label(root, text="All temperatures in degree celsius",
bg='white', font=("italic", 12))
note.place(x=90, y=485)
root.mainloop()
输出
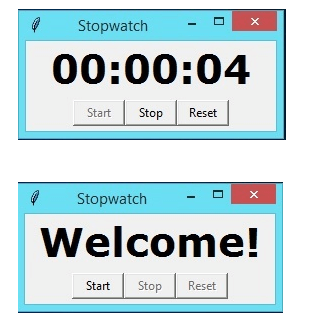